
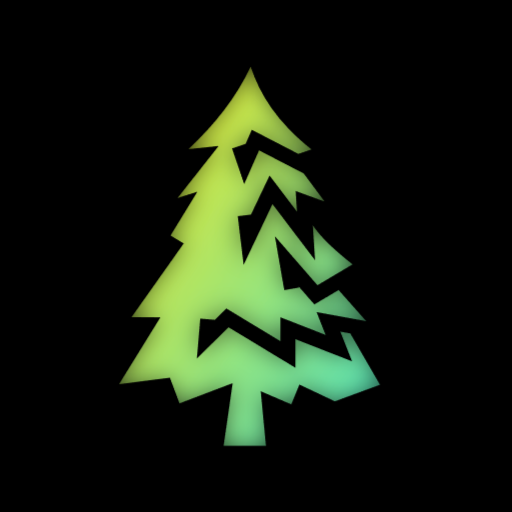
Here’s a (hopefully correct) solution (in Python) where a Five Of A Kind hand is not allowed:
Code
i = open('day7_in.txt')
from collections import Counter
card_values = {
'A': 14,
'K': 13,
'Q': 12,
'J': 0,
'T': 10
}
deques = []
for line in i:
if not line.strip():
continue
cards, bid = line.split()
cards_repr = [int(card_values.get(card, card)) for card in cards]
counts = Counter(card for card in cards if card != 'J')
deque_type = [times for card, times in counts.most_common(5)]
jokers_left = cards.count('J')
for i in range(jokers_left):
for j, n in enumerate(deque_type):
if n < 4:
deque_type[j] += 1
jokers_left -= 1
break
if jokers_left:
if jokers_left <= 4:
deque_type.append(jokers_left)
else:
deque_type += [4, 1]
deques.append((deque_type, cards_repr, int(bid), cards))
deques.sort()
ans = 0
for n, d in enumerate(deques, 1):
ans += n*d[2]
print(ans)
Oh oh, you’re correct :( How did I not notice that; guess too much thinking about standard card games, plus no hand in the example input is a Five Of A Kind.