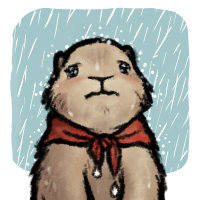
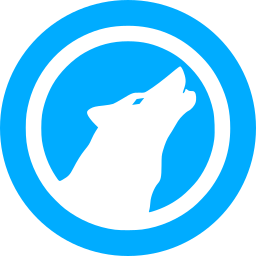
I have a similar requirement and use a self-written script in Tampermonkey, in which I falsify the wrong time (GMT) to the correct time just for websites I have previously defined. Specifically, I overwrite the newDate function on these.
This means that Librewolf always displays the wrong time (GMT) by default, except for websites I want to have the correct time (which just are a few) without the need to disable RFP.
Yes, of course. You will have to adapt it to your time zone, as the German time zone is currently hardcoded. If I had known back then that I would be sharing this at some point, I would have tried harder. 😅
// ==UserScript== // @name Spoof Timezone // @namespace http://tampermonkey.net/ // @version 0.1 // @license GPL-3.0 // @description Overwrites the Date object to use the German time zone // @author dvb // @match *://*.foo.com/* // @match *://*.bar.com/* // @match *://*.foobar.com/* // @match https://webbrowsertools.com/timezone/ // @grant none // @run-at document-start // ==/UserScript== (function() { 'use strict'; const originalDate = Date; function isSummerTime(now) { const month = now.getMonth() + 1; // January is 0, so +1 const day = now.getDate(); const hour = now.getHours(); if (month > 3 && month < 10) { // Summer time is from April to September return true; } else if (month === 3 && day >= 29 && hour >= 2) { // Last Sunday in March at 2 o'clock return true; } else if (month === 10 && day <= 25 && hour < 3) { // Last Sunday in October at 3 o'clock return true; } return false; } function getGermanDate(...args) { const now = new originalDate(...args); const germanTimeZoneOffset = isSummerTime(now) ? -120 : -60; // German time zone is UTC+1 (+2 during summer time) if (args.length === 0) { return new originalDate(now.getTime() + (now.getTimezoneOffset() - germanTimeZoneOffset) * 60000); } else { return now; } } // Overwrite the newDate function const newDate = function(...args) { return getGermanDate(...args); }; // Copy the prototypes and static methods Object.setPrototypeOf(newDate, originalDate); Object.setPrototypeOf(newDate.prototype, originalDate.prototype); for (const prop of Object.getOwnPropertyNames(originalDate)) { if (typeof originalDate[prop] === 'function') { newDate[prop] = originalDate[prop].bind(originalDate); } else { Object.defineProperty(newDate, prop, Object.getOwnPropertyDescriptor(originalDate, prop)); } } // Overwrite Date objects Date = newDate; window.Date = newDate; })();