
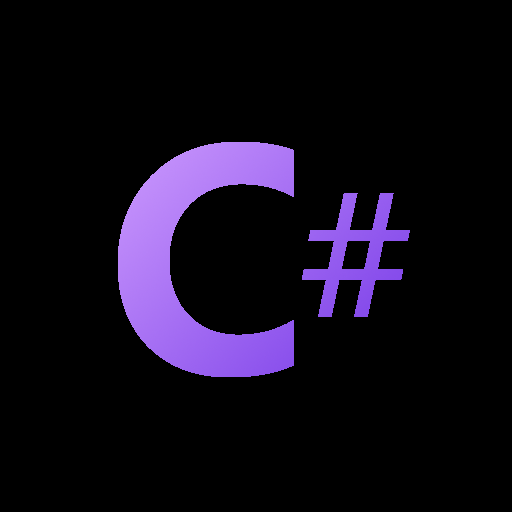
I don’t believe the first code sample is a valid C# code. Switch-case statements expect constants for case conditions and runtime types are not constants, obviously. What you can do is something like this:
void MyMethod(object arg)
{
if (arg.GetType() == typeof(int))
MyTypedMethod((int)arg);
else if (arg.GetType() == typeof(int?))
MyTypedMethod((int?)arg);
else if (arg.GetType() == typeof(long))
MyTypedMethod((long)arg);
}
This will work, but the problem here is that inheritance will fuck you up. All the types we check in this example are sealed and we don’t really have to worry about it in this case, but generally this code is… just bad.
And yes, the correct way of implementing something like this is by using generic types. Starting from C# 7.0 we have this cool structure:
void MyMethod<T>(T arg)
{
switch (arg)
{
case null:
// Do something with null
break;
case int intArg:
// Do something with intArg
break;
case long longArg:
// Do something with longArg
break;
}
}
You’ll have to check for null separately, as you can’t use Nullable<>
there, but overall, it looks much cleaner and handles inheritance properly for you.
This is called pattern matching, you can read more about it here: https://learn.microsoft.com/en-us/dotnet/csharp/language-reference/operators/patterns
Use FreeTube with VPN. But you’ll have to find a VPN provider you trust, of course. Should be a bit easier.